Tips and Tricks to Help Beginners in Unity 3D
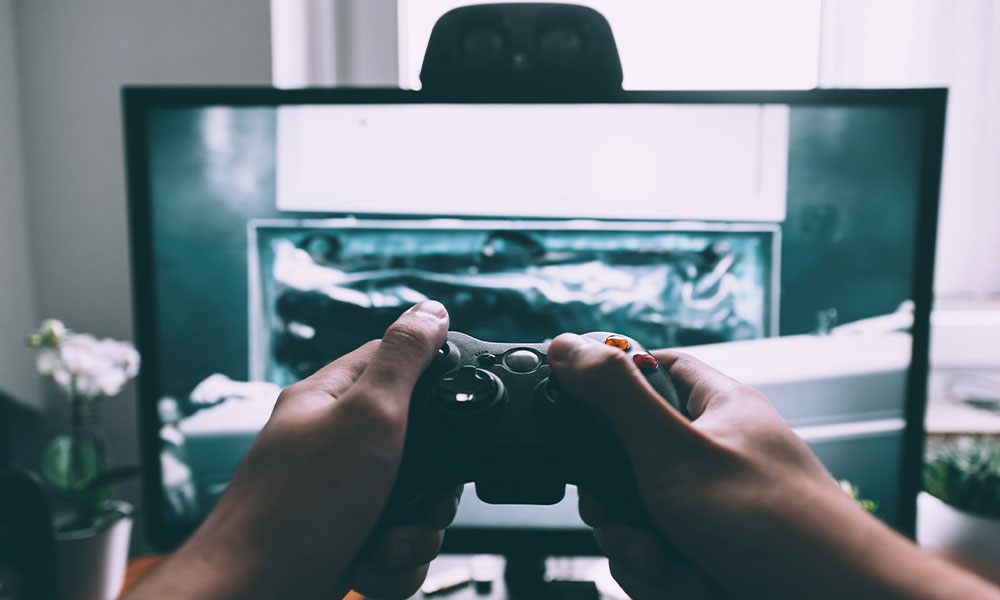
Developing games with Unity is a great way to get into the game and get started on your game development journey. The first questions a beginner asks are how to become a game developer, what to look for, and where to learn.
Don’t Use Distance () or Magnitude on Vectors
To calculate the distance between two vectors, there is a Distance () method and a magnitude field. But in the process of computing, they both use the square root operation, which is very resource-intensive. To avoid this, use the sqrMagnitude field. It returns the square of the distance between vectors. It remains only to compare it with the value, also squared.
Math Operations in Inspector Fields
In any numeric field of the inspector, you can enter not only numbers but also mathematical expressions. For example, 2/3, 0.5*7.
Since version 2021.2.0 Alpha 10, there are even more available expressions in the inspector:
- sqrt – square root extraction;
- sin / cos / tan – sine / cosine / tangent values, respectively;
- floor – rounds the value down;
- ceil – rounds the value up;
- round – rounds the value to the nearest integer.
Also, mathematical operations can be performed with the selection of several objects. For example, the expression – = 10 will subtract 10 from the corresponding coordinate of each object.
You can also distribute the coordinates randomly or equidistantly using the functions L (N, M) and R (N, M), respectively, where N and M are the minima and maximum values of the coordinates.
Rich Text in Console
Unity’s extensive customization capabilities are often overlooked in teaching game development. For example, the built-in UI text components use Rich Text formatting. It allows you to make text bold, italicized, change its color, size, and even assign material.
The same formatting is supported in the console messages displayed via Debug.Log (). For example:
Debug.Log (“<color = red> Roses are red and <color = blue> violets are blue.”);
Creating Editor Menu Items
Using the [MenuItem (“My Menu”)] attribute, you can create your own menu tabs and items. Requires import of UnityEditor space.
You can also assign hotkeys to menu items. To do this, at the end of the element name separated by a space, you need to specify one of three characters (%/#/&, which correspond to the Ctrl/Shift/Alt keys) and a letter. The main menu categories such as File, Edit, Assets, and others can also be supplemented.
Creating Additional Options in the Context Menu of a Component
In addition to your own menu items, you can create additional items in the component context menu. The [ContextMenu (“Name”)] attribute is used for this.
Saving Component Changes to Play Mode
If you make any changes to the component with the running game while creating a game in Unity, these changes will not be saved. If you made changes within one component, then all its values can be copied in the context menu of the component (Copy Component), and after you stop the game, you can paste them back through the same context menu (Paste Component Values).
If the changes were within the entire object, then in its context menu there is also the possibility of copying. But the inserted values will be the already duplicated object. So it is highly discouraged to repeat that.
Object Reference from the Message in the Console
During debugging, you may need to find the specific object that caused the error. Of course, you can display the unique ID of this object along with the message and search for it manually. But it is much easier to specify the desired object as the second argument in the Debug.Log () method. Then, when you click on a message in the console, the specified object will be highlighted in the hierarchy: Debug.Log (“Something wrong”, gameObject);
HideInInspector and SerializeField
These two attributes will help you customize the display of the desired fields in the inspector. If you have a lot of public fields that litter the inspector, then you can hide them with the HideInInspector attribute. If you need a private field that is not displayed in the inspector by default but still be editable, use the SerializeField attribute.
Automatic Depth Creation in Isometric Games
When creating a 2D game with isometric projection, you may need to simulate the depth by sorting the sprite layers. That is, the object that is in the background must be one layer below the front object. While it is easy to implement this for stationary objects by simply adjusting their layers in the SpriteRenderer component, then for moving objects it is a little more complicated.
However, Unity has already taken care of this and made it possible to sort objects by layers based on their coordinates. First, you need to set up sorting. To do this, go to Edit → Project Settings → Graphics → Camera Settings. Set the Transparency Sort Mode property to Custom Axis and the Transparency Sort Axis to (0; 1; 0). Now all objects that will be on the same layer will be automatically sorted based on the Y coordinate. Those above – to the back, and those below – to the front.
This simple trick will make developing games in Unity much easier. Indeed, without it, you would have to manually control the coordinates of various objects and change their layers.
Executing Code Not Tied to an Object
The [RuntimeInitializeOnLoadMethod (RuntimeInitializeLoadType.AfterSceneLoad)] method attribute will allow you to execute the code without binding the script to any object. In this case, the code will be called immediately after loading the scene.
Structures and classes
When developing your game, try to use structs as often as possible instead of classes. This will greatly reduce the load on the game because the structure is a value type and the class is a reference type.
Creating sublayers
In addition to ordinary layers, you can create sublayers in them, separating them with the “/” symbol. For example, Ground/Grass. This will help you classify your layers and make them easier to work with.
Focusing the Editor’s Camera on an Object and Following It
To focus the editor’s camera on an object, select that object and press F. If the object is moving and you need the camera to follow it, press F twice.
Collision Matrix
In Unity, you can define which objects will collide with each other and which will not. This is done by setting up the collision matrix. To do this, go to Edit → Project Settings → Physics → Layer Collision Matrix.
If you uncheck the box at the intersection of two layers, the objects of these layers will not collide with each other. In this case, the object of the Player layer will not collide with other objects of its own layer and objects of the Walls layer.
Debug Mode in the Inspector
It is not uncommon for errors to come across when learning to develop games. Fortunately, the standard inspector in Unity has a debug mode. It displays all the private fields of the components. To enable it, call the context menu of the inspector tab or click on the ellipsis in the upper right corner and select the Debug mode. In some situations, this mode greatly simplifies the process of debugging and developing a game.
If the component has a custom editor, then it will not be displayed in this mode – only the fields themselves will be visible there.
Monitoring via Graph
In courses on creating games, the console is often used to output values to it and monitor it. But if the value changes very often, it will not be very convenient to keep track of it. In such cases, you can plot this value on a graph. For this, you need an AnimationCurve. Sample code:
public AnimationCurve Curve = new AnimationCurve ();
private void Update ()
{
float value = Mathf.Cos (Time.time);
Curve.AddKey (Time.realtimeSinceStartup, value);
}
Object Caching
Another note from the courses on creating computer games: try to cache all the objects with which you often work. For example, in a script, you often refer to a component through GetComponent. Instead, you can create a field for this component and assign it in the Start method. After that, it will be possible to refer directly to the component through this field. This solution will reduce the load, since the operations GetComponent, Find, FindGameObjectsWithTag, etc. are resource-intensive.
Creating Presets for Components
You can create your own presets for each component. A preset allows you to save the current settings of a component and assign them to another component of the same type. Presets are stored as separate files.
To create a preset, click on the sliders icon in the upper right corner of the component. A window will open with all the presets for this type. To save the current settings, click the “Save current to…” button and save the file. In the same window, you can apply saved presets to other components.
Program Pause
Again debugging, is useful both for game developers during training and in future work. You can pause the game not only with a button in the editor but also through the code. To do this, set the EditorApplication.isPaused field to true. This can come in handy during debugging when you need to stop the game right after some error to study it in more detail.
Easily Import Files from Photoshop into Unity
By installing the 2D PSD Importer package, you can simplify the process of transferring images while developing a game from Photoshop in Unity. This package accepts files with a .psb extension. When importing such a file, it automatically creates in the object all child images that correspond to the layers from Photoshop. It also creates a group of objects with SpriteRenderer sorted by layers.
Next, you can read: When Are the Services of 3D Visualization Studio Essential?
- Dont Use Distance () or Magnitude on Vectors
- Math Operations in Inspector Fields
- Rich Text in Console
- Creating Editor Menu Items
- Creating Additional Options in the Context Menu of a Component
- Saving Component Changes to Play Mode
- Object Reference from the Message in the Console
- HideInInspector and SerializeField
- Automatic Depth Creation in Isometric Games
- Executing Code Not Tied to an Object
- Structures and classes
- Creating sublayers
- Focusing the Editors Camera on an Object and Following It
- Collision Matrix
- Debug Mode in the Inspector
- Monitoring via Graph
- Object Caching
- Creating Presets for Components
- Program Pause
- Easily Import Files from Photoshop into Unity