10 Avoidable Common Mistakes That Every Java Developer Should Know
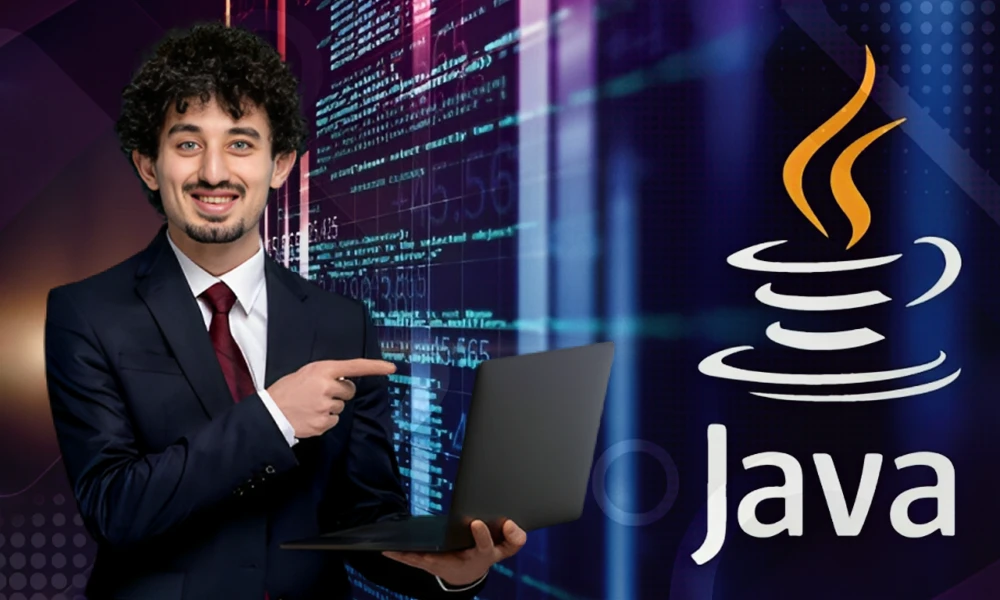
Java, a powerful and versatile programming language, is the backbone of countless applications and software solutions. However, even the most seasoned Java developers sometimes fall into common pitfalls that hinder their progress.
Hiring a Java developer is essential for software development due to Java’s versatility, platform independence, and robustness. Java developers specialize in building scalable and secure applications, making them valuable for creating web apps, mobile solutions, big data technologies, and enterprise-level systems.
A Java Development Services Company offers diverse possibilities, enabling businesses to innovate in various domains and making it a top choice for building reliable and future-proof software applications.
In this blog post, we’ll explore ten of these mistakes, shedding light on why they occur and how to avoid them while you look for developers for projects like developing mobile app development with Java. Let’s dive in!
- Lack of Planning: One of developers’ most common mistakes is diving into coding without a solid plan. Without a clear roadmap, your code can quickly become convoluted and complex. Take the time to outline your approach, consider different scenarios, and design a robust structure before you start coding.
Correction – Plan your code structure and logic before coding.
Example: Create a flowchart or pseudocode outlining the steps before writing Java code.
- Memorization Over Understanding: Relying on memory instead of understanding the underlying concepts can lead to mistakes. Instead of memorizing code snippets, focus on comprehending their logic and principles. This way, you can apply your knowledge to various situations and write more efficient and maintainable code.
Correction – Focus on understanding concepts, not memorizing code.
Example: Learn the logic behind sorting algorithms instead of memorizing sorting code snippets.
- Misusing Data Types: Using raw instead of parameterized types is a standard error. Parameterized types provide type safety, ensuring you work with the appropriate data types. Neglecting this practice can lead to unexpected behavior and errors in your code.
Correction – Use parameterized types for type safety.
Example: Instead of List list = new ArrayList();, use List<String> list = new ArrayList<>();.
- Ignoring Existing Libraries: Java boasts many libraries that can simplify complex tasks. Neglecting these libraries and reinventing the wheel can waste time and effort. Explore existing libraries and leverage their functionalities to enhance code quality and productivity.
Explore and utilize relevant libraries for specific tasks.
Example: Use Apache Commons IO library for file operations instead of creating custom methods.
- Overlooking Compile-Time Errors: Compile-time errors are your best friends; they highlight potential issues before your code runs. Ignoring these errors or bypassing them can result in runtime disasters. Please pay close attention to compiler warnings and errors, addressing them promptly for a robust codebase.
Address compiler warnings and errors promptly.
Example: Correct syntax errors highlighted by the compiler before running the program.
- Handling Null Pointers Ineffectively: Null pointer exceptions can haunt Java developers. Failing to handle null pointers gracefully can crash your application. Always validate objects for null before accessing their methods or properties to prevent these dreaded exceptions.
Validate objects for null before accessing methods or properties.Example: Instead of object.method(), use if (object != null) { object.method(); }.
- Resource Management: Forgetting to release resources like file handles, database connections, or network sockets can lead to resource leaks. Always use try-with-resources or finally blocks to ensure proper resource management, preventing memory leaks and improving the overall efficiency of your code.
Use try-with-resources or finally blocks for proper resource release.
Example: try (FileInputStream fis = new FileInputStream(“file.txt”)) { /* code */ }.
- Ignoring Design Patterns: Design patterns are essential tools for Java developers. They provide proven solutions to recurring design problems. Neglecting these patterns can lead to inefficient and error-prone code. Please familiarize yourself with design patterns and apply them where appropriate to create robust and maintainable software.
Apply appropriate design patterns for efficient solutions.
Example: Use the Singleton pattern for a class, ensuring only one instance exists.
- Handling Exceptions Wisely: Ignoring or handling exceptions improperly can result in unexpected program behavior or crashes. Always handle exceptions gracefully, log relevant information, and provide meaningful error messages to users. Understanding exception types and their appropriate handling techniques is crucial for Java developers.
Handle exceptions gracefully with meaningful messages.
Example:
try {
// code that may throw an exception
} catch (Exception e) {
System.err.println(“An error occurred: ” + e.getMessage());
}
10. Understanding Control Flow: Misusing control flow statements, such as forgetting the ‘break’ keyword in a switch-case block, can lead to unintended fall-through behavior. Additionally, accessing non-static variables from static methods can cause inconsistencies. Always understand the flow of control in your code, ensuring that each statement is correctly placed and serves its intended purpose.
Use the ‘break’ keyword in the switch case and avoid accessing non-static variables from static methods.
Example:
switch (variable) {
Case 1:
// code
break;
Default:
// code
break;
}
public static void staticMethod() {
// code, but avoid accessing non-static variables here
}
Conclusion –
Be aware of these common mistakes and actively hire Java developers who can significantly enhance the quality and reliability of the code. Continuous learning, attention to detail, and a proactive approach to problem-solving are crucial to finding a proficient Java developer.